TRAINING: Custom LUA Plugins
1.) Preparations
You need:
- Notepad++ or any other coding / text editor
- Baneto + Unlocker
- Wow Account
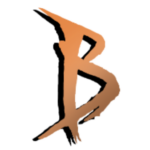
2.) Hello World plugin
A) Open Notepad++
B) “Language” Tab, set it to L – LUA
C) Write following code:
print(“Hello World”)
D) Save it to scripts/baneto/plugins folder as HelloWorld.lua
E) Open the game and (re)load baneto. Enable the bot setting “Baneto Plugins”
Explanation:
The print function takes the input (arguments) and outputs it in your ingame chat. This is NOT visible for anyone else. ONLY your client can see it.
3.) Bunny Hopper Plugin
A) Open Notepad++
B) “Language” Tab, set it to L – LUA
C) Write following code:
BANETO_JumpOrAscendStart()
D) Save it to scripts/baneto/plugins folder as BunnyHopper.lua
E) Open the game and (re)load baneto. Enable the bot setting “Baneto Plugins”
Explanation:
Remember how we were able to put something into the print(“text”) function? The BANETO_JumpOrAscendStart() function does not take any input (arguments). When executed (called) the function will make the bot jump once. Since baneto plugins are loop executed this plugin will make him jump literally forever.
Info:
The BANETO_JumpOrAscendStart() function and many more can be found in our baneto api doc
You can also find many native wow lua functions in wow’s lua api
4.) Advanced Bunny Hopper Plugin
Task:
Our current bunny hopper plugin constantly jumps and never stops. Now we will change it to only jump every 10 seconds.
A) Open Notepad++
B) “Language” Tab, set it to L – LUA
C) Write following code:
if not bunny_jumpDelay or bunny_jumpDelay < GetTime() then
bunny_jumpDelay = GetTime()+10
BANETO_JumpOrAscendStart()
end
D) Save it to scripts/baneto/plugins folder as BunnyHopper.lua (overwrite the old one)
E) Open the game and (re)load baneto. Enable the bot setting “Baneto Plugins”
Explanation:
The BANETO_JumpOrAscendStart() function is now encapsulated by an if statement that checks whether GetTime() is greater or less than the timestamp variable bunny_jumpDelay. Everytime the jump is executed we set the timestamp to 10 seconds in the future.
Extra Task:
Add a print(“we are jumping now”) every time the jump happens. It is important to distinguish from it spamming multiple times per second (wrong) or only once per 10 seconds perfectly synced with each jump (correct).
5.) Use item on low life or low mana
Task:
We want to use an item if we are low on health or low on mana. Both cases will trigger the usage of different items. An example would be health and mana potions.
A) Open Notepad++
B) “Language” Tab, set it to L – LUA
C) Write following code:
local health = BANETO_UnitHealth(“player”)
local mana = BANETO_UnitMana(“player”)
if health < 30 then
BANETO_UseItem(1234)
end
if mana < 20 then
BANETO_UseItem(5678)
end
D) Save it to scripts/baneto/plugins folder as UsingItemsOnLowHpOrMp.lua
E) Open the game and (re)load baneto. Enable the bot setting “Baneto Plugins”
Explanation:
The item id’s 1234 and 5678 are placeholders. You can find item ids, object ids and unit ids on Wowhead
We are constantly checking the health and save it in our variable health.
We are constantly checking the mana and save it in our variable mana.
Then we compare the saved variables with hardcoded values (30% for health in this case, and 20% for mana in this case)
As soon as health drops below 30% or mana drops below 20% it will trigger the code inside the if statements and use the items. (1234 for health below 30 and 5678 for mana below 20)
Extra Task:
Encapsulate this entire code with a lua delay like in 4.) to make sure that the usage of an item can only be triggered once every 10 minutes, even if the health or mana drops below the tresholds.
6.) Tips & Tricks
Documentation:
Learn to read and interpret / test documentations like found in our baneto api doc
Test functions via ingame chat:
/dump BANETO_JumpOrAscendStart()
/dump BANETO_UnitHealth(“player”)
Add prints everywhere:
print(“DEBUG A”)
if BANETO_UnitHealth(“player”) < 20 then
print(“DEBUG B”)
end
This can help you understand the flow of your code at runtime.